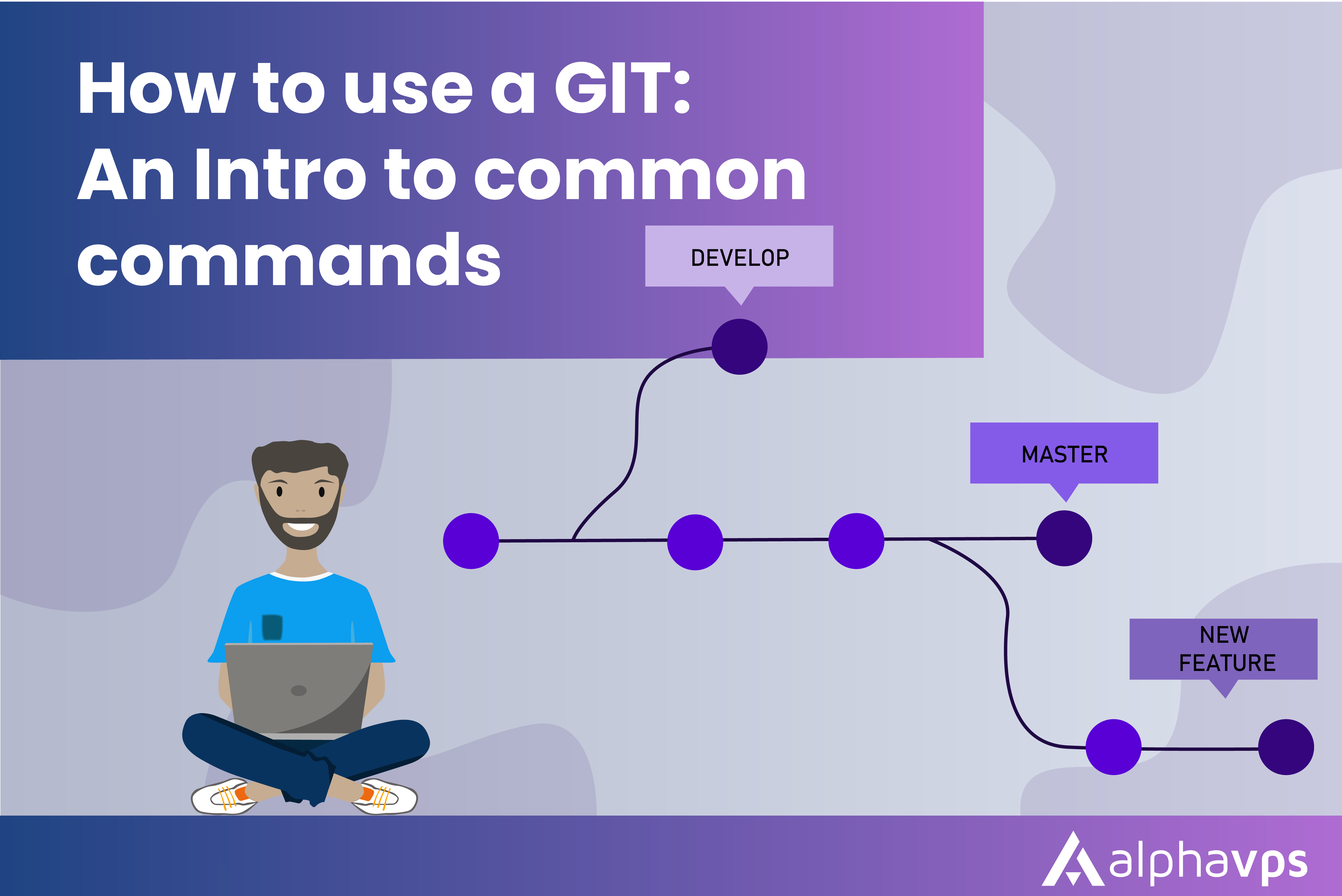
GIT is a widely used distributed version control system that allows a team of developers to work on the same project simultaneously. This allows them to collaborate on a project efficiently.
This tutorial serves as a continuation of our Git tutorial here.
Consider it as a reference to common Git commands, which are useful for working with a Git repository.
Initialization
Initialize a new Git repository in a directory. When you run git init
in a directory, it sets up the necessary data structures and files needed for Git to track changes to your project.
git init
Copy an existing repository from a remote host. You need to specify the remote repository's URL. For example, https://www.github.com/your-username/repository
git clone <url>.
To check your current directory's remote repository, you can run:
git remote
Add a remote repository to your local Git repository. Provide a name, which will serve as a label for the remote repository, and its URL.
git remote add <name> <url>
Staging
You can check the status of your Git repository by running:
git status
To include modified files in our next commit, first, we will need to stage them. This can be done with the git add
command.
To add a specific file, we need to run:
git add <file1.txt>
We can also specify multiple files by separating them with a comma.
git add <file1.txt>,<file2.js>,<file3.html>,<file4.py>
If you want to add all files in the current directory, simply run:
git add .
Remove a file from staging by running:
git reset <file1.txt>
Commiting
Staged files can be committed to our local repository, making the changes to them permanent. To do this, we can run the following command:
git commit -m "your commit message here"
-m
option and a commit message, you will be prompted to do this immediately after the command with your default text editor. Commit messages need to be short, concise, and with just enough information about the performed additions or changes. It is recommended that your commits are well planned and do not include changes that are not related.If we need to modify our commit message, we can run:
git commit --amend -m "updated commit message"
Branches
Branches are a feature that allows parallel development and isolated work on a project. A branch represents an independent line of development within a Git repository, enabling you to make changes without affecting the main project or other branches like it.
You can list your current branch by running:
git branch
Your active branch will have an asterisk *
next to it.
You can create a new branch by running:
git branch <new-branch-name>
Switch your current working directory to another existing branch:
git checkout <branch-name>
The two previous commands can be combined by running:
git checkout -b <new-branch-name>
This command will create a new branch and directly switch your current directory to it.
You can also rename your branch by running:
git branch -m <old-branch-name> <new-branch-name>
Merging branches
Merging branches is the process of combining changes from one branch into another. When branches are merged, Git combines their commit histories and content. This does not create an entirely new branch.
The branch you merge into is referred to as the "target branch" - it remains the same. The changes from the other branch, referred to as the "source branch", are integrated into the target branch. After the merge is complete, you continue using the target branch.
You can merge your current working branch with another specified branch by running:
git merge <branch-name>
Merges can be aborted in case of conflicts.
git merge --abort
Instead of merging entire branches, we can only "cherry-pick" specific commits from them and merge them to our target branch. For this, we will need the hash pointing to the required commit from the source branch.
We can check the commit logs for a specific branch by running:
git log <branch-name>
More convenient formatting of the output can be added by running:
git log <branch-name> --oneline
You will receive an output with a similar structure:
c53234d (HEAD -> main) Add feature XYZ
a9f9c3e Fix bug ABC
f02de7b Update documentation
The first string on each line is actually a pointer to its respective commit.
Grab the required hash /or hashes, if you want to merge multiple commits/ and run:
git cherry-pick <hash>
Once a branch is no longer required, after merging it you are able to delete it with:
git branch -d <branch-name>
You can also forcefully delete it by running
git branch -D <branch-name>
Collaborating with remote repositories
Fetch the current state of the remote branch, which you are currently working on, and merge its commits with your local repository.
git pull
Push your local commits to the default remote repository and branch configured for the current local branch.
git push
You can also specify the remote repository and its branch.
git push origin main
Git history
You are able to view the current branch's commit history by running:
git log
To review only the commits for a specific file, you can run:
git log --follow <file1.html>
You are able to review changes between commits by running:
git diff <commit-1> <commit2>
You will need to provide the pointer of the required commits.
To review the difference between branches, you can run:
git diff <branch1> <branch2>
Reverting changes
You can revert back the changes, which were applied by a specific commit. To do this you will need to grab the required commit's pointer/hash and run the following command:
git revert <commit>
Ignoring files
If you have files, which are kept in your local Git directory, but do not want to be committed and pushed to your remote repository, you can specify them in your .gitignore
file.
You can create a .gitignore
file by running touch .gitignore
and providing the relative paths to the files that need to be ignored.
For example, the node-modules
folder of a NodeJS project is ignored due to its larger size.